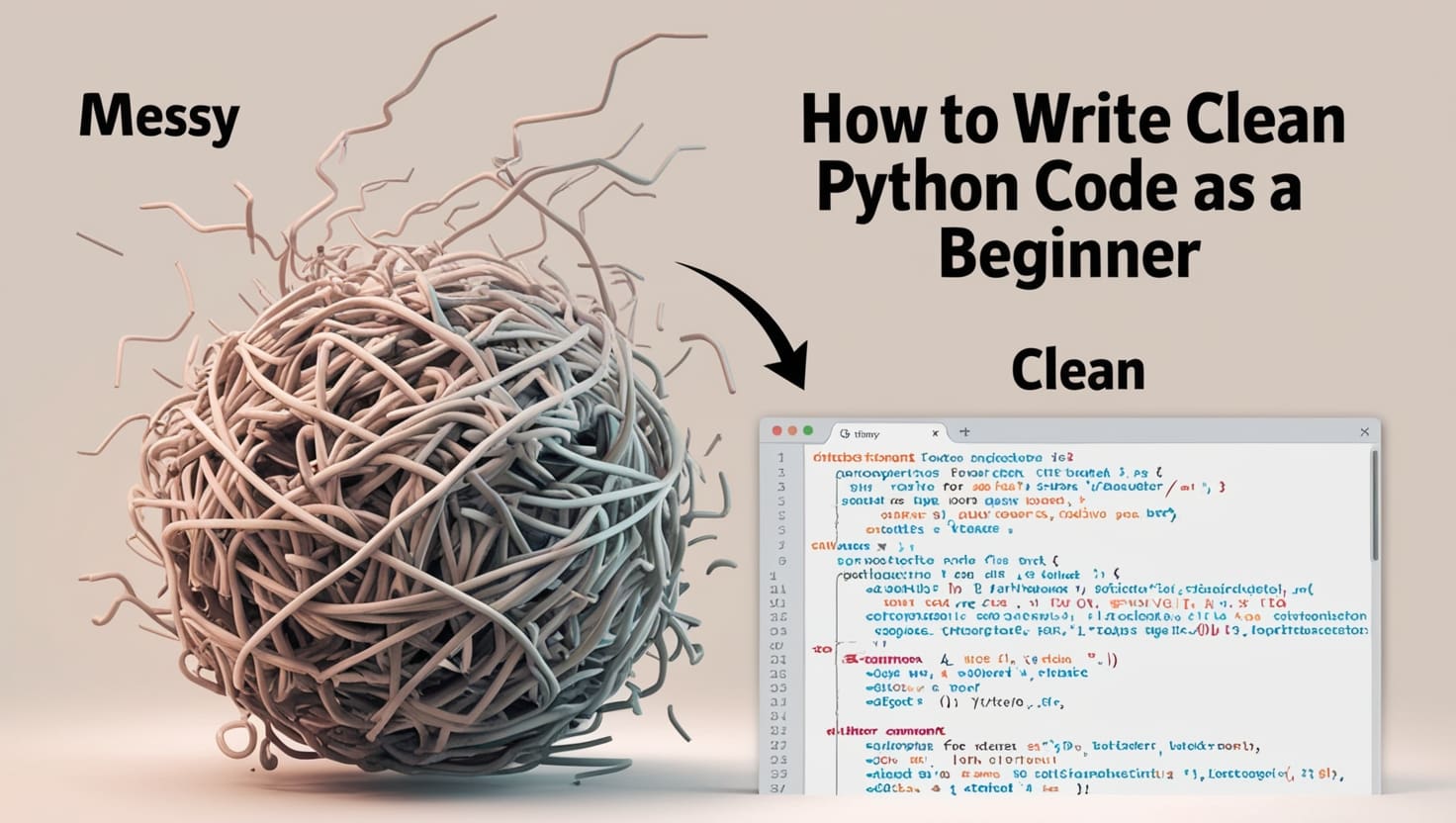
Image by Author| Leonardo.ai
Python is a great beginner language—simple and clear. But as you get more comfortable with it, it’s easy to slip into the bad habit of writing convoluted, unreadable code that is a developer’s worst nightmare to debug. Clean code isn’t just about making it pleasant to look for someone else—it’ll save you a ton of headaches when you have to deal with it again. Take my word on this, taking the time to write neat code will make your own life a hell of a lot easier in the long run.
In this article, we will look at some tips and best practices that will enable you to write clean and readable Python code even if you are a beginner.
Key Practices for Writing Clean, Beginner-Friendly Python Code
1. Follow the PEP 8 Style Guide
PEP 8 is the main style guide for Python, and it provides conventions for writing clean and readable code. Here are a few important points made in PEP 8:
- Use 4 spaces per indentation level:
- Limit line length to 79 characters: Keep your code readable by not writing overly long lines. If a line gets too long, break it into smaller parts using parentheses or backslashes.
- Use blank lines to separate code blocks: Add blank lines between functions or classes to improve readability:
- Use snake_case for variable and function names: Variables and functions should be lowercase with words separated by underscores:
def greet():
print("Hello, World!") # Indented with 4 spaces
def add(a, b):
return a + b
def subtract(a, b):
return a - b
my_variable = 10
def calculate_average(numbers):
return sum(numbers) / len(numbers)
2. Use Meaningful Names for Variables and Functions
Don’t use vague or one-letter names (like `x` or `y` if it is not for simple and short loops. This isn’t useful to the code. Instead, give names that explain what the variable or the function does.
- Bad Example:
- Good Example:
def a(x, y):
return x + y
def add_numbers(first_number, second_number):
return first_number + second_number
3. Use Clear Comments (But Don’t Overdo It)
Comments justify why your code does something but not what it does. If your codebase is clean and does not contain vague naming, you wouldn’t need to comment as much. The code should, in principle, speak for itself! However, when necessary, use comments to clarify your intentions.
4. Keep Functions Short and Focused
A function should do one thing and do it well. If a function is too long or handles multiple tasks, consider breaking it into smaller functions.
5. Handle Errors Gracefully
As a beginner, it’s tempting to skip error handling, but it’s an important part of writing good code. Use try and except blocks to handle potential errors.
try:
number = int(input("Enter a number: "))
print(f"The number is number")
except ValueError:
print("That’s not a valid number!")
This ensures your program doesn’t crash unexpectedly.
6. Avoid Hardcoding Values
Hardcoding values (e.g., numbers or strings) directly into your code can make it difficult to update or reuse. Instead, use variables or constants.
- Bad Example:
- Good Example:
print("The total price with tax is: $105") # Hardcoded tax and total price
PRICE = 100 # Base price of the product
TAX_RATE = 0.05 # 5% tax rate
# Calculate the total price
total_price = PRICE + (PRICE * TAX_RATE)
print(f"The total price with tax is: $total_price:.2f")
This makes your code more flexible and easy to modify.
7. Avoid Global Variables
Relying on global variables can make your code harder to understand and debug. Instead, encapsulate state within classes or functions.
- Bad Example (using a global variable):
- Good Example (using a class):
total = 0
def add_to_total(value):
global total
total += value
class Calculator:
def __init__(self):
self.total = 0
def add_value(self, value):
self.total += value
Encapsulating data within objects or functions ensures that your code is modular, testable, and less error-prone.
8. Use f-Strings for String Formatting
f-Strings (introduced in Python 3.6) are a clean and readable way to format strings.
- Bad Example (concatenating strings):
- Good Example (using f-strings):
name = "Alice"
age = 25
print("My name is " + name + " and I am " + str(age) + " years old")
name = "Alice"
age = 25
print(f"My name is name and I am age years old")
f-Strings are not only more readable but also more efficient than other string formatting methods.
9. Use Built-in Functions and Libraries
Python comes with many powerful built-in features. Use these to write efficient and proper code instead of coding it from scratch.
- Bad Example (manually finding the maximum):
- Good Example (using max):
def find_max(numbers):
max_number = numbers[0]
for num in numbers:
if num > max_number:
max_number = num
return max_number
def find_max(numbers):
return max(numbers)
10. Use Pythonic Code
“Pythonic” code refers to writing code that takes advantage of Python’s simplicity and readability. Avoid overly complex or verbose solutions when simpler options are available.
- Bad Example:
- Good Example:
numbers = [1, 2, 3, 4, 5]
doubled = []
for num in numbers:
doubled.append(num * 2)
numbers = [1, 2, 3, 4, 5]
doubled = [num * 2 for num in numbers]
Using list comprehensions, built-in functions, and readable idioms makes your code more elegant.
11. Use Version Control
Even as a beginner, it’s a good idea to start using tools like Git for version control. It allows you to track changes, collaborate with others, and avoid losing progress if something goes wrong.
Learn the basics of Git:
- Save your code with git add and git commit
- Experiment without fear, knowing you can revert to a previous version.
12. Structure Your Project Well
As your codebase grows, organizing your files and directories becomes essential. A well-structured project makes it easier to navigate, debug, and scale your code.
Here is an example of a standard project structure:
my_project/
├── README.md # Project documentation
├── requirements.txt # Project dependencies
├── setup.py # Package configuration for distribution
├── .gitignore # Git ignore file
├── src/ # Main source code directory
│ └── my_project/ # Your package directory
│ ├── __init__.py # Makes the folder a package
│ ├── main.py # Main application file
│ ├── config.py # Configuration settings
│ └── constants.py # Project constants
├── tests/ # Test files
│ ├── __init__.py
│ ├── test_main.py
│ └── test_utils.py
├── docs/ # Documentation files
│ ├── api.md
│ └── user_guide.md
└── scripts/ # Utility scripts
└── setup_db.py
A structured approach ensures your project remains clean and manageable as it grows in size.
13. Test Your Code
Always test your code to ensure it works as expected. Even simple scripts can benefit from testing. For automated testing, beginners can start with the built-in unittest module.
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add(self):
self.assertEqual(add_numbers(2, 3), 5)
self.assertEqual(add_numbers(-1, 1), 0)
if __name__ == "__main__":
unittest.main()
Testing helps you catch bugs early and ensures your code works correctly.
Remember:
- Write code for humans, not just computers
- Keep it simple
- Stay consistent with your style
- Test your code regularly
- Refactor when needed
Wrapping Up
Do it little by little, keep on learning, and soon clean code will be your second nature. This article is for complete Python newbies. To learn advanced strategies on how to write better maintainable code in Python, read my article: Mastering Python: 7 Strategies For Writing Clear, Organized, and Efficient Code
Kanwal Mehreen Kanwal is a machine learning engineer and a technical writer with a profound passion for data science and the intersection of AI with medicine. She co-authored the ebook “Maximizing Productivity with ChatGPT”. As a Google Generation Scholar 2022 for APAC, she champions diversity and academic excellence. She’s also recognized as a Teradata Diversity in Tech Scholar, Mitacs Globalink Research Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having founded FEMCodes to empower women in STEM fields.