Agent Development Kit (ADK) is an open-source Python framework that helps developers build, manage, and deploy multi-agent systems. It’s designed to be modular and flexible, making it easy to use for both simple and complex agent-based applications.
In this tutorial, we’ll create a simple AI agent using ADK. The agent will have access to two tools:
- get_company_overview
- get_earnings
Google API Key
To use Google’s AI services, you’ll need an API key:
AlphaVantage API Key
For accessing financial data, we’ll use the Alpha Vantage API:
- Go to https://www.alphavantage.co/
- Click “Get your free API key” or visit this direct link
- Enter your email and follow the instructions
- Once you receive your API key, copy and save it securely. We’ll use it to authenticate requests to financial endpoints.
Python Libraries
We only need one package:
Set up your project folder with the following structure:
parent_folder/
│
└───multi_agent/
├── __init__.py
├── agent.py
└── .env
__init__.py
Paste the following code into multi_agent/__init__.py:
.env
Create a .env file inside the multi_agent folder and paste the following:
GOOGLE_GENAI_USE_VERTEXAI=FALSE
GOOGLE_API_KEY="<YOUR_GOOGLE_API_KEY>"
ALPHA_VANTAGE_API_KEY="<YOUR_ALPHA_VANTAGE_KEY"
Replace the placeholders with your actual API keys
agent.py
Paste the following code in the agent.py file:
from google.adk.agents import Agent
import requests
import os
from typing import Optional
ALPHA_VANTAGE_API_KEY = os.getenv("ALPHA_VANTAGE_API_KEY")
def get_company_overview(symbol: str) -> dict:
"""
Get comprehensive company information and financial metrics
Args:
symbol: Stock ticker symbol (e.g., IBM)
Returns:
dict: Company overview data or error
"""
if not ALPHA_VANTAGE_API_KEY:
return {"status": "error", "error": "Missing API key"}
base_url = "https://www.alphavantage.co/query"
params = {
"function": "OVERVIEW",
"symbol": symbol,
"apikey": ALPHA_VANTAGE_API_KEY
}
try:
response = requests.get(base_url, params=params)
response.raise_for_status()
data = response.json()
if "Error Message" in data:
return {"status": "error", "error": data["Error Message"]}
# Filter key metrics
key_metrics = {
"Description": data.get("Description"),
"Sector": data.get("Sector"),
"MarketCap": data.get("MarketCapitalization"),
"PERatio": data.get("PERatio"),
"ProfitMargin": data.get("ProfitMargin"),
"52WeekHigh": data.get("52WeekHigh"),
"52WeekLow": data.get("52WeekLow")
}
return {
"status": "success",
"symbol": symbol,
"overview": key_metrics
}
except Exception as e:
return {"status": "error", "error": str(e)}
def get_earnings(symbol: str) -> dict:
"""
Get annual and quarterly earnings (EPS) data with analyst estimates and surprises
Args:
symbol: Stock ticker symbol (e.g., IBM)
Returns:
dict: Earnings data with estimates or error message
"""
if not ALPHA_VANTAGE_API_KEY:
return {"status": "error", "error": "Missing API key"}
base_url = "https://www.alphavantage.co/query"
params = {
"function": "EARNINGS",
"symbol": symbol,
"apikey": ALPHA_VANTAGE_API_KEY
}
try:
response = requests.get(base_url, params=params)
response.raise_for_status()
data = response.json()
if "Error Message" in data:
return {"status": "error", "error": data["Error Message"]}
# Process annual and quarterly earnings
annual_earnings = data.get("annualEarnings", [])[:5] # Last 5 years
quarterly_earnings = data.get("quarterlyEarnings", [])[:4] # Last 4 quarters
# Format surprise percentages
for q in quarterly_earnings:
if "surprisePercentage" in q:
q["surprise"] = f"{q['surprisePercentage']}%"
return {
"status": "success",
"symbol": symbol,
"annual_earnings": annual_earnings,
"quarterly_earnings": quarterly_earnings,
"metrics": {
"latest_eps": quarterly_earnings[0]["reportedEPS"] if quarterly_earnings else None
}
}
except Exception as e:
return {"status": "error", "error": str(e)}
root_agent = Agent(
name="Financial_analyst_agent",
model="gemini-2.0-flash",
description=(
"Agent to give company overviews with key financial metrics."
),
instruction=(
"You are a helpful AI agent that provides company overviews and earnings information"
),
tools=[get_company_overview, get_earnings],
)
In this script, we define a financial analysis agent using the Google Agent Development Kit (ADK). The agent is designed to answer user queries by accessing real-time financial data through the Alpha Vantage API. Specifically, it exposes two tools: get_company_overview and get_earnings. The get_company_overview function retrieves key company details such as sector, market capitalization, P/E ratio, and 52-week high/low values. The get_earnings function provides both annual and quarterly earnings data, including reported EPS and surprise percentages.To create the agent, we use the Agent class from the google.adk.agents module, giving it a name, a model (e.g., Gemini 2.0 Flash), a description, and an instruction prompt. The agent is then equipped with the two tools mentioned above, allowing it to respond to questions related to company financials.
To run the agent, navigate to the parent directory of your agent project (e.g. using cd ..)
parent_folder/ ← Navigate to this directory in your terminal
│
└───multi_agent/
├── __init__.py # Initializes the module
├── agent.py # Contains the agent logic and tools
└── .env # Stores your API keys securely
After navigating, run the following code:
Open the URL provided (usually http://localhost:8000 or http://127.0.0.1:8000) directly in your browser. You’ll see a simple chat interface where you can interact with your agent using the input textbox.
Additionally, you can inspect each step of the agent’s reasoning by clicking on Actions. This allows you to view:
- The tools being called
- The inputs and outputs of each function
- The responses generated by the language model
You can find the entire code along with folder structure at this link: https://github.com/mohd-arham-islam/ADK-demo
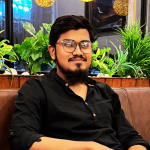
I am a Civil Engineering Graduate (2022) from Jamia Millia Islamia, New Delhi, and I have a keen interest in Data Science, especially Neural Networks and their application in various areas.