Extracting and analyzing stock data is key to informed decision-making in the financial landscape. This tutorial offers a comprehensive guide to building an integrated financial analysis and reporting tool in Python. We will learn to pull historical market data from Yahoo Finance and compute essential technical indicators such as Simple Moving Averages, Bollinger Bands, MACD, and RSI. The guide walks you through generating insightful visualizations and compiling them into custom multi-page PDF reports. Whether you are a data enthusiast, financial analyst, or Python developer looking to expand your toolkit, this tutorial will equip you with skills that help turn raw market data into actionable insights.
import yfinance as yf
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.backends.backend_pdf import PdfPages
We import essential Python libraries for financial data analysis and visualization. Here, yfinance is used for fetching stock market data, pandas for data manipulation, matplotlib and numpy for creating and handling numerical plots, and PdfPages for compiling multiple plots into a single PDF report.
def compute_indicators(df):
"""
Computes technical indicators for the DataFrame:
- 20-day and 50-day Simple Moving Averages (SMA)
- Bollinger Bands (using 20-day SMA ±2 standard deviations)
- MACD (12-day EMA minus 26-day EMA) and its 9-day Signal Line
- RSI (Relative Strength Index) over a 14-day lookback period
"""
df['SMA20'] = df['Close'].rolling(window=20).mean()
df['SMA50'] = df['Close'].rolling(window=50).mean()
df['STD20'] = df['Close'].rolling(window=20).std()
df['UpperBand'] = df['SMA20'] + 2 * df['STD20']
df['LowerBand'] = df['SMA20'] - 2 * df['STD20']
df['EMA12'] = df['Close'].ewm(span=12, adjust=False).mean()
df['EMA26'] = df['Close'].ewm(span=26, adjust=False).mean()
df['MACD'] = df['EMA12'] - df['EMA26']
df['Signal'] = df['MACD'].ewm(span=9, adjust=False).mean()
delta = df['Close'].diff()
gain = delta.copy()
loss = delta.copy()
gain[gain < 0] = 0
loss[loss > 0] = 0
loss = loss.abs()
avg_gain = gain.rolling(window=14).mean()
avg_loss = loss.rolling(window=14).mean()
rs = avg_gain / avg_loss
df['RSI'] = 100 - (100 / (1 + rs))
return df
This function computes key technical indicators—including SMAs, Bollinger Bands, MACD, and RSI—for stock price data contained in the input DataFrame. It updates the DataFrame with additional columns for each indicator, enabling in-depth technical analysis of historical stock performance.
def create_cover_page(pdf):
"""
Creates and saves a cover page into the PDF report.
"""
fig = plt.figure(figsize=(11.69, 8.27))
plt.axis('off')
plt.text(0.5, 0.7, "Financial Analysis Report", fontsize=24, ha="center")
plt.text(0.5, 0.62, "Analysis of 5 Stocks from Yahoo Finance", fontsize=16, ha="center")
plt.text(0.5, 0.5, "Includes Technical Indicators: SMA, Bollinger Bands, MACD, RSI", fontsize=12, ha="center")
plt.text(0.5, 0.4, "Generated with Python and matplotlib", fontsize=10, ha="center")
pdf.savefig(fig)
plt.close(fig)
This function creates a visually appealing cover page using matplotlib and adds it as the first page of the PDF report via the provided PdfPages object. It then closes the figure to free up resources.
def plot_price_chart(ticker, df):
"""
Generates a price chart with SMA and Bollinger Bands for a given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 7))
ax.plot(df.index, df['Close'], label="Close Price", linewidth=1.5)
ax.plot(df.index, df['SMA20'], label="SMA (20)", linewidth=1.2)
ax.plot(df.index, df['SMA50'], label="SMA (50)", linewidth=1.2)
ax.plot(df.index, df['UpperBand'], label="Upper Bollinger Band", linestyle="--")
ax.plot(df.index, df['LowerBand'], label="Lower Bollinger Band", linestyle="--")
ax.fill_between(df.index, df['LowerBand'], df['UpperBand'], color="lightgray", alpha=0.3)
ax.set_title(f'{ticker}: Price & Moving Averages with Bollinger Bands')
ax.set_xlabel('Date')
ax.set_ylabel('Price')
ax.legend()
ax.grid(True)
return fig
This function generates a comprehensive stock price chart for a given ticker that includes the close price, 20-day and 50-day SMAs, and the Bollinger Bands. It returns a matplotlib figure that can be saved or further processed in a PDF report.
def plot_macd_chart(ticker, df):
"""
Generates a MACD plot for the given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 5))
ax.plot(df.index, df['MACD'], label="MACD", linewidth=1.5)
ax.plot(df.index, df['Signal'], label="Signal Line", linewidth=1.5)
ax.set_title(f'{ticker}: MACD')
ax.set_xlabel('Date')
ax.set_ylabel('MACD')
ax.legend()
ax.grid(True)
return fig
This function generates a MACD chart for a specified ticker by plotting the MACD and its Signal Line over time. It returns a matplotlib figure that can be incorporated into a larger PDF report or displayed independently.
def plot_rsi_chart(ticker, df):
"""
Generates an RSI plot for the given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 5))
ax.plot(df.index, df['RSI'], label="RSI", linewidth=1.5)
ax.axhline(70, color="red", linestyle="--", linewidth=1, label="Overbought (70)")
ax.axhline(30, color="green", linestyle="--", linewidth=1, label="Oversold (30)")
ax.set_title(f'{ticker}: RSI')
ax.set_xlabel('Date')
ax.set_ylabel('RSI')
ax.legend()
ax.grid(True)
return fig
This function generates an RSI chart for a given stock ticker, plotting the RSI values along with horizontal reference lines at the overbought (70) and oversold (30) levels. It returns a matplotlib figure that can be incorporated into the final financial analysis report.
def main():
tickers = []
for i in range(5):
ticker = input(f"Enter ticker #{i+1}: ").upper().strip()
tickers.append(ticker)
pdf_filename = "financial_report.pdf"
with PdfPages(pdf_filename) as pdf:
create_cover_page(pdf)
for ticker in tickers:
print(f"Downloading data for {ticker} from Yahoo Finance...")
df = yf.download(ticker, period='1y')
if df.empty:
print(f"No data found for {ticker}. Skipping to the next ticker.")
continue
df = compute_indicators(df)
fig_price = plot_price_chart(ticker, df)
pdf.savefig(fig_price)
plt.close(fig_price)
fig_macd = plot_macd_chart(ticker, df)
pdf.savefig(fig_macd)
plt.close(fig_macd)
fig_rsi = plot_rsi_chart(ticker, df)
pdf.savefig(fig_rsi)
plt.close(fig_rsi)
print(f"PDF report generated and saved as '{pdf_filename}'.")
Here, this main function prompts the user to input five stock tickers, downloads one year of data for each from Yahoo Finance, computes key technical indicators, and generates corresponding price, MACD, and RSI charts. It then compiles all the charts into a multi‐page PDF report named “financial_report.pdf” and prints a confirmation message once the report is saved.
if __name__ == "__main__":
main()
Finally, this block checks whether the script is executed directly rather than imported as a module. If so, it calls the main() function.
In conclusion, we have demonstrated a method for automating financial analysis using Python. You have learned how to extract valuable data, calculate key technical indicators, and generate comprehensive visual reports in a multi-page PDF format. This integrated approach streamlines the analysis process and provides a powerful way to visualize market trends and monitor stock performance. As you further customize and expand upon this framework, you can continue to enhance your analytical capabilities and make more informed financial decisions.
Here is the Colab Notebook. Also, don’t forget to follow us on Twitter and join our Telegram Channel and LinkedIn Group. Don’t Forget to join our 85k+ ML SubReddit.
🔥 [Register Now] miniCON Virtual Conference on AGENTIC AI: FREE REGISTRATION + Certificate of Attendance + 4 Hour Short Event (May 21, 9 am- 1 pm PST) + Hands on Workshop
Sana Hassan, a consulting intern at Marktechpost and dual-degree student at IIT Madras, is passionate about applying technology and AI to address real-world challenges. With a keen interest in solving practical problems, he brings a fresh perspective to the intersection of AI and real-life solutions.
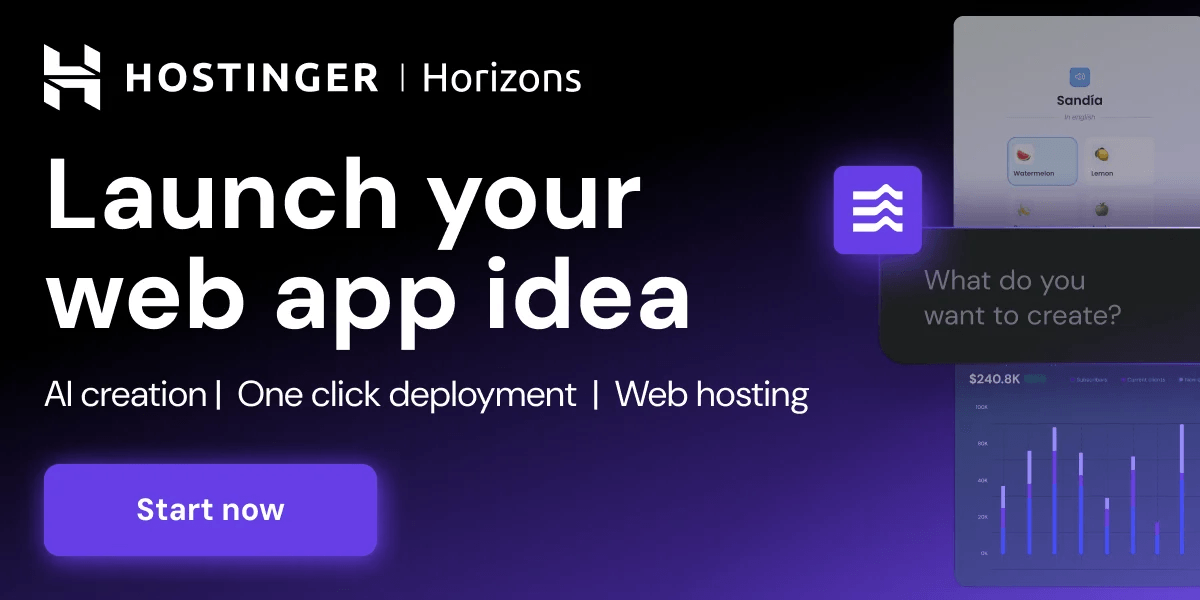