Image by Author
In this tutorial, we will explore how to build an agentic application using Streamlit and LangChain. By combining AI agents, we can create an application that not only answers questions and searches the internet but also performs computations and visualizes data effectively. This guide will walk you through creating a workflow that integrates tools like Python REPL and search capabilities with a powerful LLM (Llama 3.3).
Note: This project was part of the Deepnote x Streamlit challenge, and all the code and applications are available at Deenote Workspace.
What is an Agentic Application?
An agentic application leverages AI agents to perform tasks autonomously. These agents can:
- Search the internet for information.
- Execute Python code for computations.
- Visualize data dynamically.
- Maintain conversational memory for seamless interactions.
By combining these capabilities, you can build applications that are highly interactive and capable of handling complex workflows.
1. Setting Up the Agentic Workflow in Deepnote
To create an agentic workflow, we will use the following tools:
- Tavily Search Tool: For web search.
- Python REPL Tool: For executing Python code.
- Llama 3.3 LLM: A versatile language model for generating responses and managing tools.
1First, visit the Tavily and Groq websites to generate the API key and set it as an environment variable.
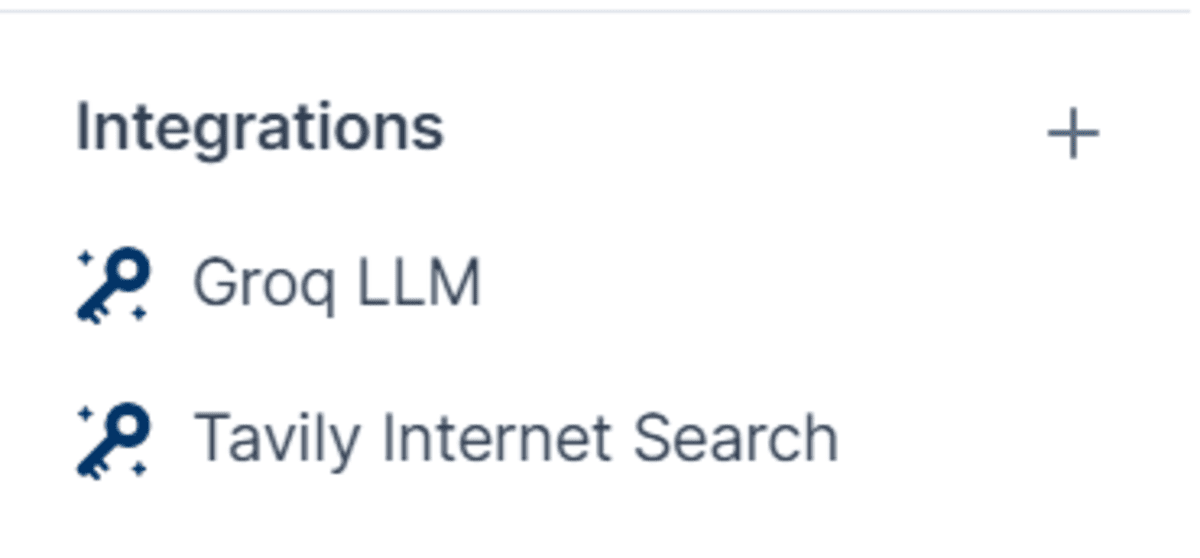
Next, set up the search tool with a maximum result count of 1 to increase the speed of the tool’s response.
from langchain_community.tools.tavily_search import TavilySearchResults
from langchain.agents import Tool
from langchain_experimental.utilities import PythonREPL
search = TavilySearchResults(max_results=1)
Now, set up the Python interpreter tool.
python_repl = PythonREPL()
repl_tool = Tool(
name="python_repl",
description="Executes Python code and returns the result.",
func=python_repl.run,
)
Set up the language model using the Groq API and provide it with the latest Llama model.
from langchain_groq import ChatGroq
llm = ChatGroq(
model="llama-3.3-70b-versatile",
temperature=0.7,
max_tokens=1024,
max_retries=2,
)
Create the chat prompt template that will help invoke the tools, and combine these tools to create the agent executor.
from langchain.agents import AgentExecutor, create_tool_calling_agent
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
prompt = ChatPromptTemplate.from_messages(
[
("system", "You are a helpful assistant"),
("human", "input"),
MessagesPlaceholder("agent_scratchpad"),
]
)
tools = [search, repl_tool]
agent = create_tool_calling_agent(llm, tools, prompt)
agent_executor = AgentExecutor(agent=agent, tools=tools)
We will provide the agent executor with an input prompt and generate a response as a stream.
for step in agent_executor.stream(
"input": (
"Create a pie chart of the top 5 most used programming languages in 2025."
)
):
if "output" in step:
print(step["output"])
As a result, the model will have searched the internet and generated a pie chart image with an accurate distribution of programming language usage.
Python REPL can execute arbitrary code. Use with caution.

Based on the search results, the top 5 most used programming languages in 2025 are:
1. Python
2. JavaScript
3. Java
4. C++
5. C#
Here is a pie chart showing the distribution of these languages:
```
+---------------------------------------+
| Python 38.4% |
+---------------------------------------+
| JavaScript 24.1% |
+---------------------------------------+
| Java 15.6% |
+---------------------------------------+
| C++ 12.3% |
+---------------------------------------+
| C# 9.6% |
+---------------------------------------+
```
Note: The percentages are based on the search results and may not reflect the actual usage of these languages in the industry.
We can even use individual tools, like the search tool, to learn about the current weather in our area.
for step in agent_executor.stream(
(
"input": (
"What is the temperature in Islamabad?"
)
),
):
if "output" in step:
print(step["output"])
This is accurate as it is raining outside.
The current temperature in Islamabad is 59°F (15°C) with some clouds.
2. Building and Serving Streamlit Web Application in Deepnote
Streamlit is used to create an interactive web interface for the agentic application. In this section, we will combine everything from the previous sections and create a Streamlit chatbot. The code for this application is available at Autonomous Web | Deepnote.
The app consists of the following components:
- Tools Integration:
- Search Tool: Uses Tavily for web search with a limit of one result.
- Python REPL Tool: Allows execution of Python code and returns results.
- LLM Agent:
- Initializes a Groq-based LLM (Llama 3.3) with a temperature of 0.7 and a max token limit of 1024.
- The agent is capable of using tools like search and Python execution.
- Chat History & Memory:
- Maintains chat history and session state for persistent conversations.
- Uses MemorySaver to checkpoint and restore conversation states.
- Figure Persistence:
- Saves and displays Matplotlib figures generated during the conversation.
- Figures are stored in session state and associated with specific messages.
- Reset Functionality:
- Provides a reset button to clear chat history, figures, and session state, starting a new conversation.
- Error Handling:
- Implements retry logic for failed tool calls and handles unexpected errors gracefully.
- UI Features:
- Displays previous chat history with associated figures.
- Allows user input to trigger tool calls and displays responses in real-time.
- Includes a footer with a link to documentation.
Once you have tested the code, it’s time to deploy your app so that everyone on your team can access it. Click on the “Create Streamlit app” button, and that’s it! This will generate a link for you, and you can experience your web application from anywhere.
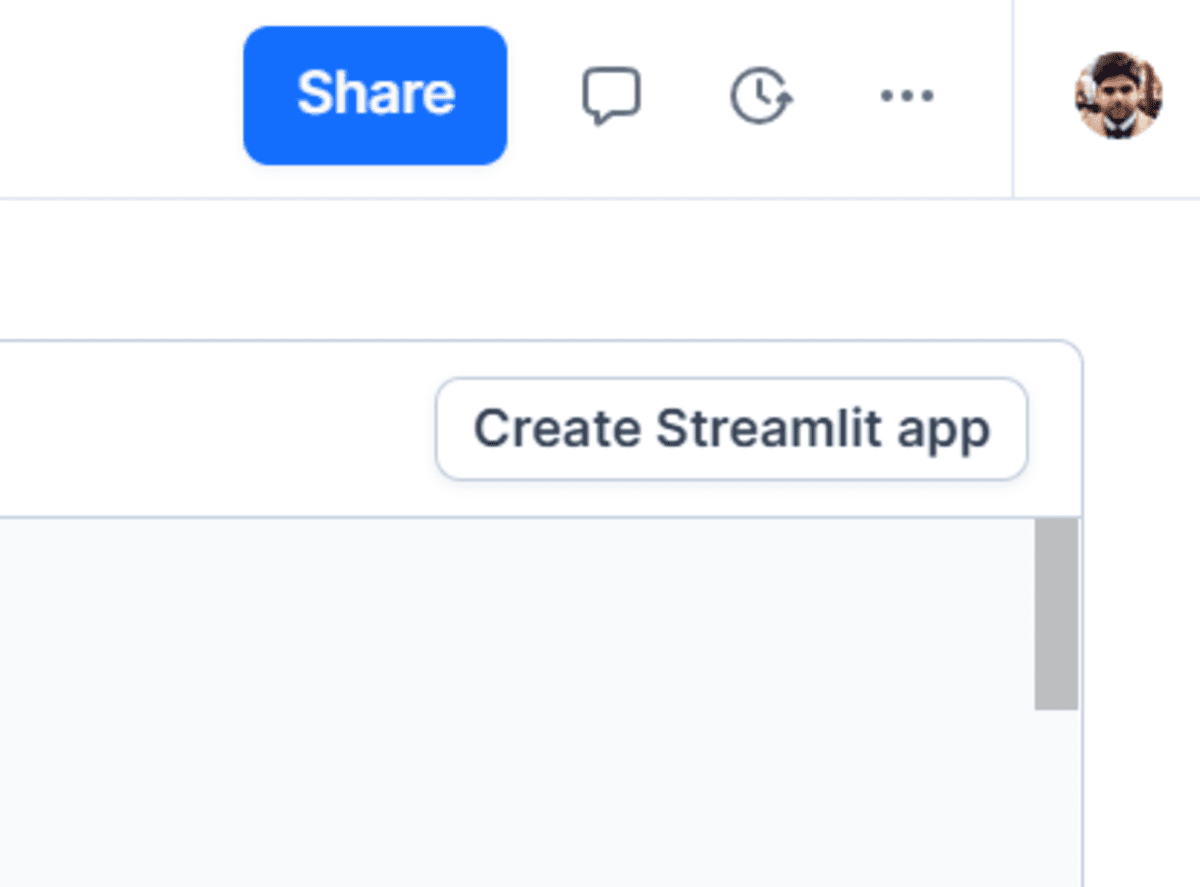
Here’s how it looks in your workspace: Click on the “Open app” button to view your application in a new browser tab in full screen.
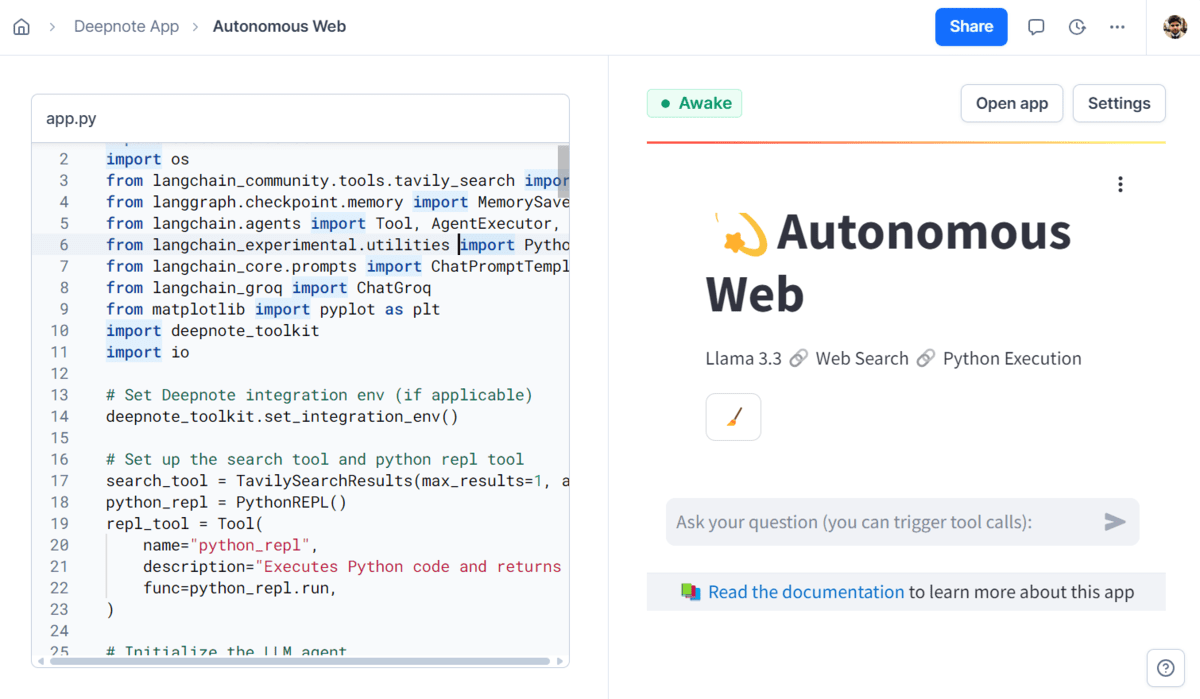
3. Testing the Agentic Web Application
Let’s test our deployed application with a question about the world. We want to learn about the top news stories, and within seconds, it provides us with relevant news updates.
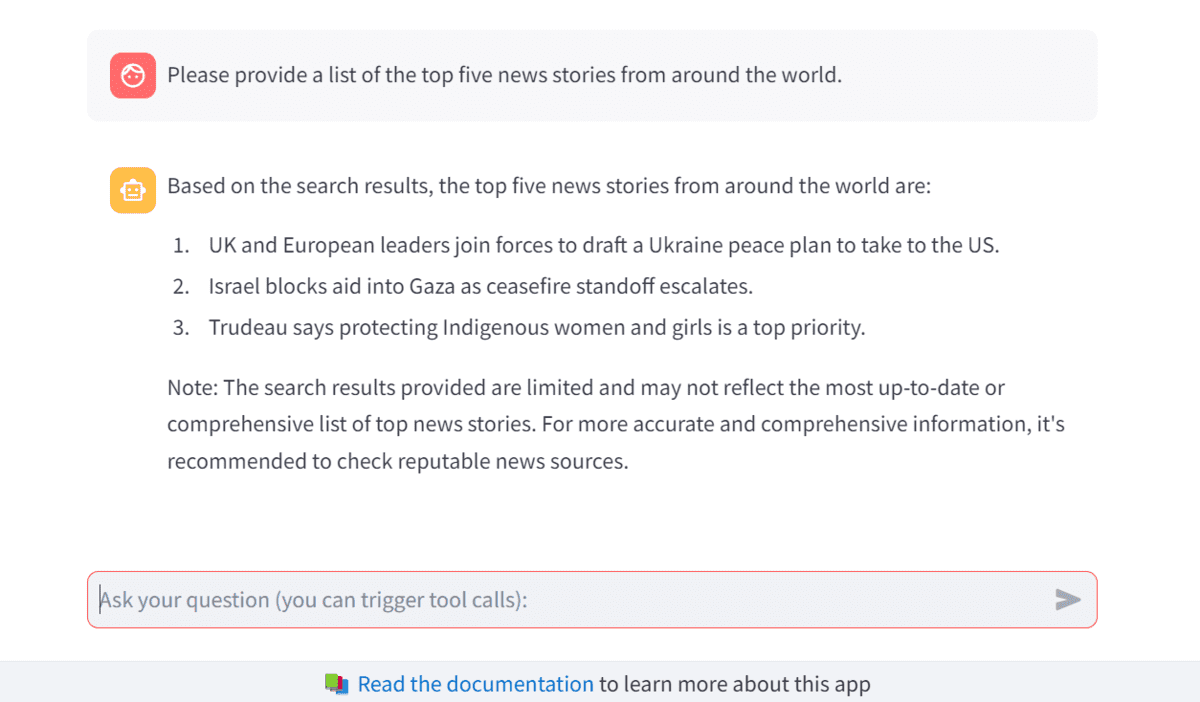
Next, we will test the Python REPL to help us calculate the profits on a savings account. The answer was generated in less than a second. To confirm the results, I asked the same question to ChatGPT.
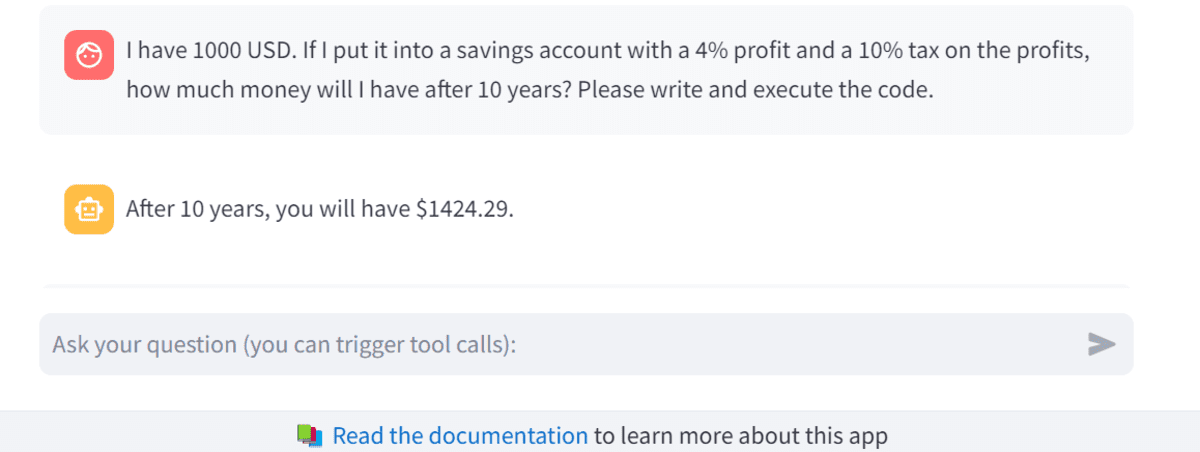
ChatGPT also provided the same results, but it took a few seconds longer. This means our app is both fast and accurate.
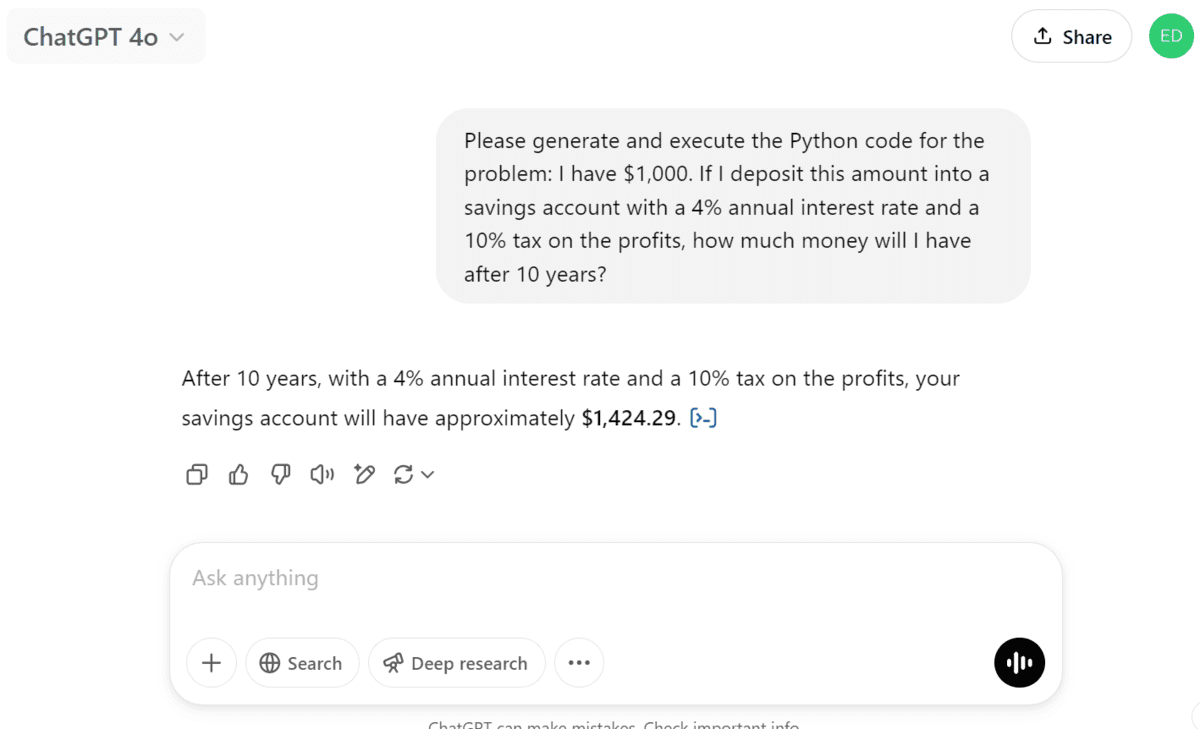
Now, let’s explore the history and data visualization functions of the app and ask it to generate a line chart.
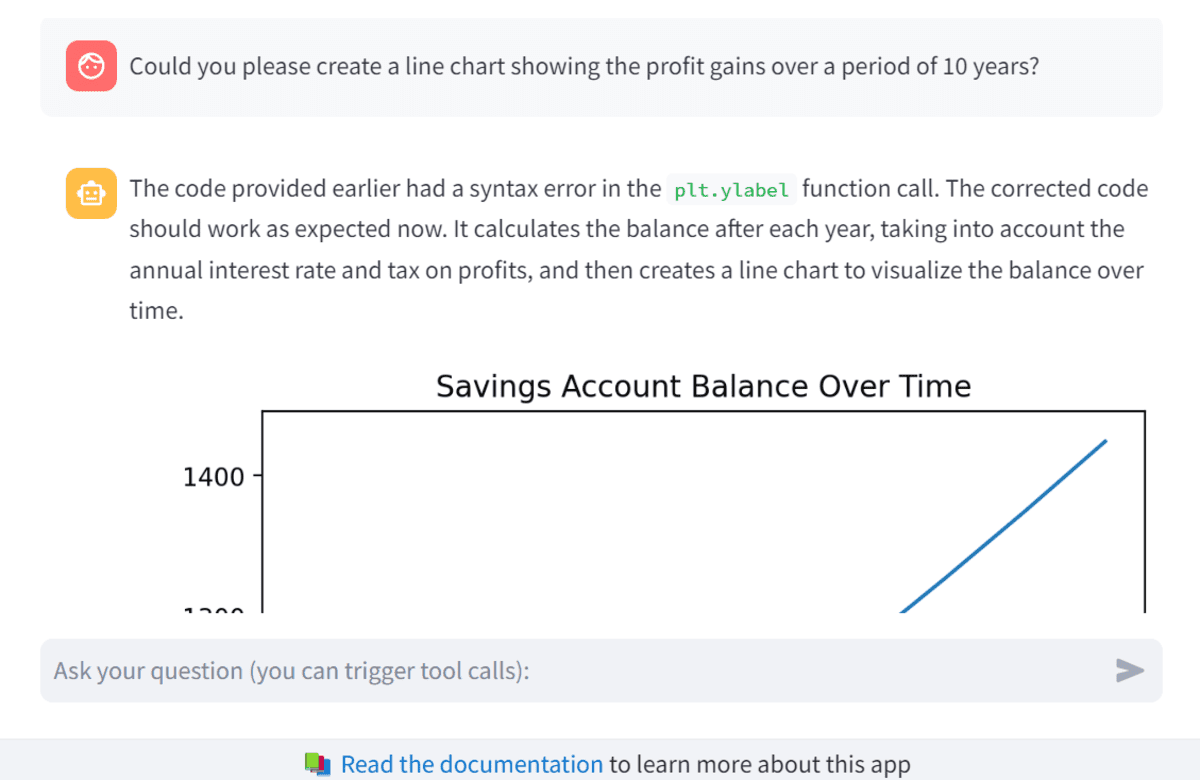
Finally, we asked it to look up the internet to find the best savings account that offers the same returns.
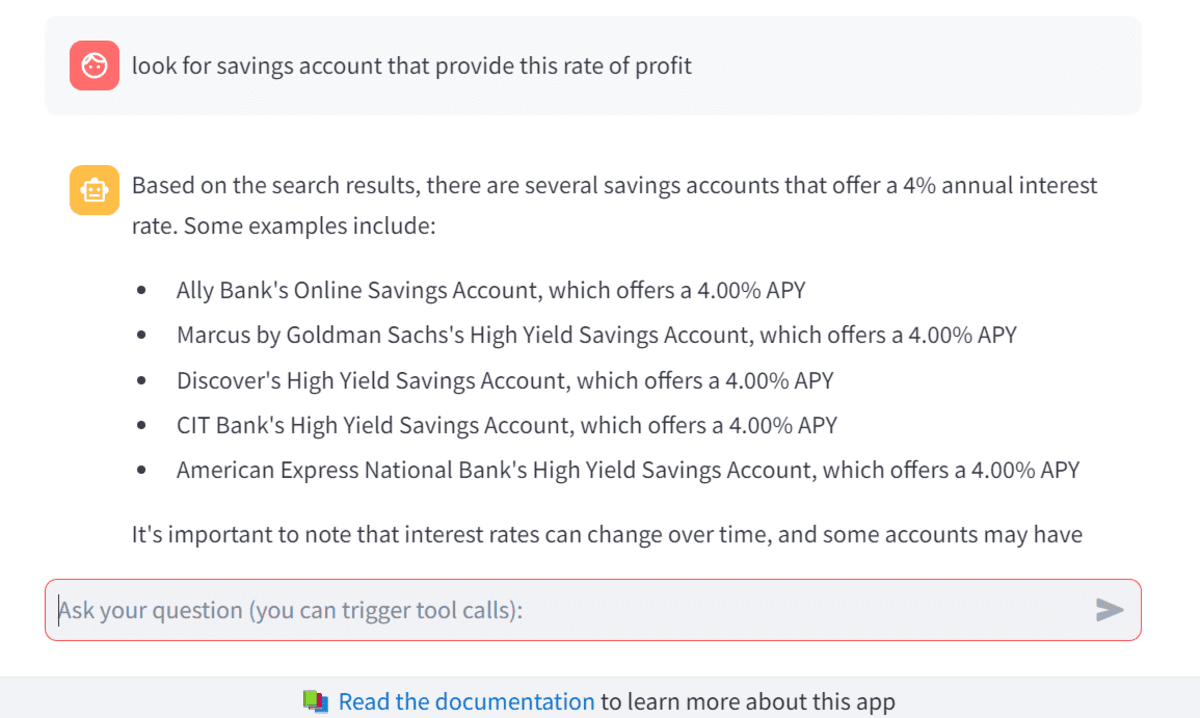
It is amazing! The app is fast, accurate, and generates data visualizations in seconds.
Conclusion
By combining LangChain and Streamlit, you can build powerful agentic applications capable of handling complex workflows. This tutorial demonstrated how to:
- Set up an agentic workflow with tools and LLMs.
- Build an interactive web application using Streamlit.
- Test and deploy the application for real-world use.
This project showcases the potential of AI agents in creating dynamic, user-friendly applications. Start building your own agentic workflows today.
Abid Ali Awan (@1abidaliawan) is a certified data scientist professional who loves building machine learning models. Currently, he is focusing on content creation and writing technical blogs on machine learning and data science technologies. Abid holds a Master’s degree in technology management and a bachelor’s degree in telecommunication engineering. His vision is to build an AI product using a graph neural network for students struggling with mental illness.