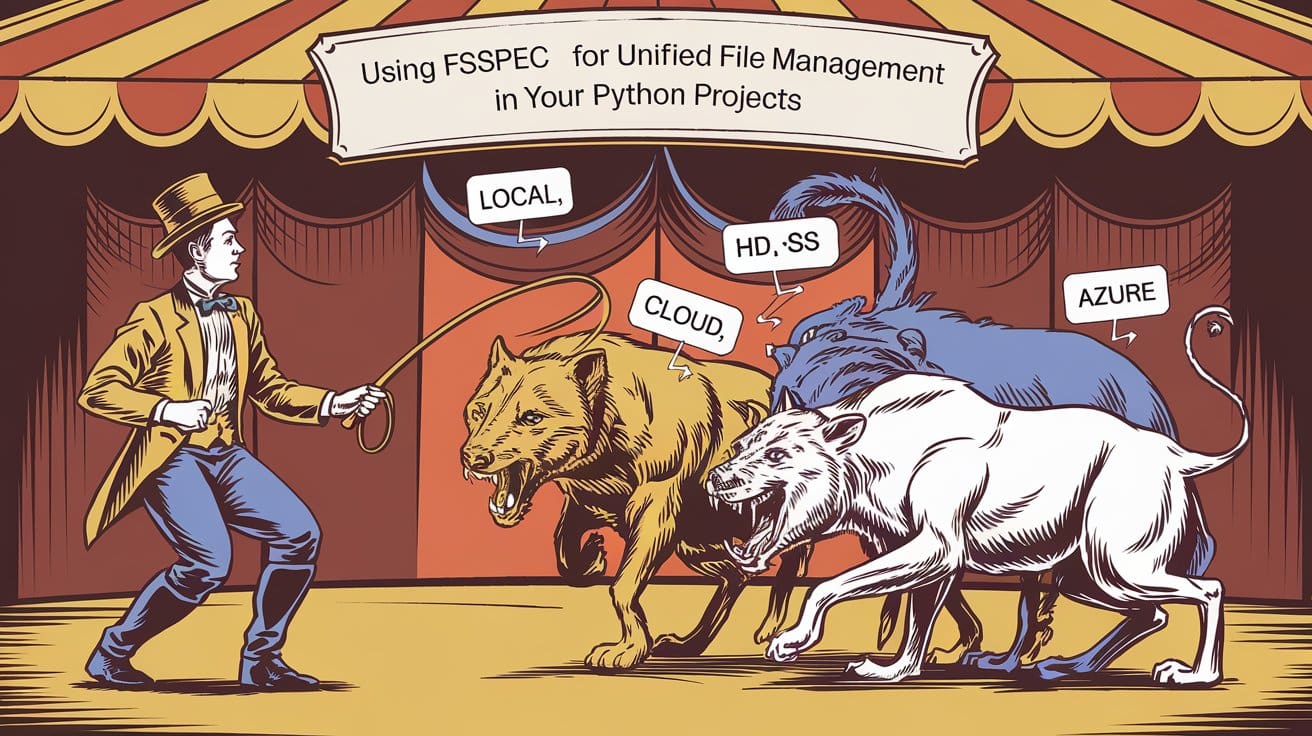
Image by Editor (Kanwal Mehreen) | Ideogram.ai
Â
Managing files across different systems can be complex, especially in data science, machine learning, and web development. Files may be stored locally on your machine, in cloud services, or on remote servers. Each system often requires a different set of tools and APIs to interact with the files. This can lead to complicated code and slower workflows.
fsspec is a Python library that simplifies this process. It provides a single interface for accessing and managing files from all these different storage systems. With fsspec, you can use the same code to work with files stored on your computer, cloud services like AWS S3 or Google Cloud, and remote systems such as FTP and SFTP.
Â
Key Features of fsspec
Â
- Unified File System Interface: Use the same commands for files stored on your computer, in the cloud, or on remote servers.
- Support for Multiple Storage Backends: Work with files in AWS S3, Google Cloud, Azure Blob, HDFS, FTP, and SFTP without extra tools.
- Caching and Performance Optimization: Make file access faster by storing files locally after the first time you access them
- Streaming Large Files: Work with large files without loading all the data into memory at once, , which helps avoid memory problems when handling big files.
- Glob Patterns for File Discovery: Search for files quickly by using special patterns (like wildcards) to match file names.
Â
Installing fsspec
Â
You can install fsspec using pip:
Â
If you need additional support for specific storage backends (e.g., AWS S3, Google Cloud Storage), you can install extra dependencies:
pip install fsspec[aws] # For AWS S3 support
pip install fsspec[gcs] # For Google Cloud Storage support
Â
Basic Usage of fsspec
Â
Here’s how you can start using fsspec to manage files:
Â
1. Accessing Local Files
Accessing local files with fsspec is simple. You can use the open function to read and write files on your local system. By specifying the ‘file’ backend, fsspec treats local files as if they were in remote storage. This makes it easier to switch between local and remote file systems without changing the code structure.
import fsspec
# Open a local file
fs = fsspec.filesystem('file')
with fs.open('local_file.txt', 'r') as f:
data = f.read()
print(data)
Â
2. Accessing Cloud Files
fsspec makes it easy to work with cloud storage, such as AWS S3. To access files on S3, you need to install the s3fs dependency. After connecting to S3 with your credentials, you can read and write files as if they were stored locally.
import fsspec
# Connect to AWS S3
fs = fsspec.filesystem('s3', key='your-access-key', secret="your-secret-key")
# Read a file from S3
with fs.open('s3://bucket-name/file.txt', 'r') as f:
data = f.read()
print(data)
Â
3. Working with Remote Files
fsspec also supports remote file systems such as FTP and SFTP. You can open and work with files stored on remote servers, just like you would with local files. You need to specify the remote system and provide the necessary connection details (host, username, password).
# For FTP
fs = fsspec.filesystem('ftp', host="ftp.server.com", username="user", password='password')
# Open a file over FTP
with fs.open('/remote/path/to/file.txt', 'r') as f:
data = f.read()
print(data)
# For SFTP (Similar process)
fs = fsspec.filesystem('sftp', host="sftp.server.com", username="user", password='password')
with fs.open('/remote/path/to/file.txt', 'r') as f:
data = f.read()
print(data)
Â
4. In-Memory Files
fsspec lets you to work with files stored directly in memory. This can be useful when dealing with small datasets or when you don’t need to interact with physical storage. You can use the ‘memory’ backend to treat data as a file without reading or writing to disk.
# Use in-memory file system
fs = fsspec.filesystem('memory')
# Write data to in-memory file
with fs.open('myfile.txt', 'w') as f:
f.write('This is some text')
# Read from in-memory file
with fs.open('myfile.txt', 'r') as f:
data = f.read()
print(data)
Â
Advanced Features of fsspec
Â
1. Caching and Performance Optimization
fsspec improves performance by caching files. It stores files locally after the first access. This reduces the need to re-download files. Caching speeds up file handling. It also lowers network overhead.
fs = fsspec.filesystem('s3', cache_storage="/path/to/cache")
with fs.open('s3://bucket-name/file.txt', 'r') as f:
data = f.read()
Â
2. Glob Patterns and Directory Listing
fsspec supports glob patterns to list files in a directory. You can use wildcard characters to match files. This is helpful when working with multiple files. It’s useful for datasets spread across several files.
# List all text files in an S3 bucket
fs = fsspec.filesystem('s3')
files = fs.glob('s3://bucket-name/*.txt')
print(files)
Â
3. Parallel Operations with Dask
fsspec works with Dask for parallel operations on large datasets. Dask enables distributed computing for large-scale data processing. Combining fsspec and Dask lets you load and process remote data. This is great for working with data stored in cloud storage.
import dask.dataframe as dd
import fsspec
# Use fsspec to read from a cloud storage and load into Dask DataFrame
fs = fsspec.filesystem('s3')
ddf = dd.read_csv('s3://bucket-name/*.csv', storage_options='client': fs)
Â
Conclusion
Â
fsspec is a useful Python library for managing files across different systems. It provides an easy and consistent way to work with local, remote, and cloud storage. With features like caching, glob patterns, and large file streaming, fsspec makes file management faster and more efficient. You can even use it with Dask to process large datasets in parallel. Start using fsspec today to simplify your file management workflows and unlock the full potential of your Python projects!
Â
Â
Jayita Gulati is a machine learning enthusiast and technical writer driven by her passion for building machine learning models. She holds a Master’s degree in Computer Science from the University of Liverpool.