When Matsuoka’s WebGPU-powered water simulations started making waves on X, they amazed developers and graphics enthusiasts alike with their breathtaking realism and incredible performance. These simulations aren’t just beautiful—they are a showcase of what’s possible with WebGPU. Naturally, we had to know more. So, we invited him to share the techniques behind these mesmerizing simulations and how WebGPU is pushing the boundaries of what’s possible in the browser!
Over the past few months, I have implemented two fluid simulations using WebGPU: WebGPU-Ocean and WaterBall. In this article, I would like to share some insights and techniques I gained while implementing them.
While developing these two simulations, I consistently focused on two key aspects:
- The simulation must be highly performant.
- My goal is to implement large-scale fluid simulations that run smoothly in browsers across as many devices as possible. They must perform efficiently on laptops with integrated graphics (iGPU), and ideally, they should also run well on lower-end devices, such as my six-year-old iPad Air 3!
- The visualization must be visually appealing.
- Even if the simulation performs exceptionally well, poor visualization can completely ruin the experience. Therefore, I need to carefully select and implement a rendering method that enhances the fluid’s visual appeal.
Looking back, I can confidently say that these goals have been successfully achieved! The first was accomplished using MLS-MPM (Moving Least Squares Material Point Method) implemented with WebGPU, while the second was achieved through a rendering technique called Screen-Space Fluid Rendering. In this article, I will provide a detailed explanation of these methods.
Quick Tour of My Projects
Before diving into the details of fluid simulation, let’s briefly review the two fluid simulations I have implemented so far.
The first project is WebGPU-Ocean (GitHub Repository):
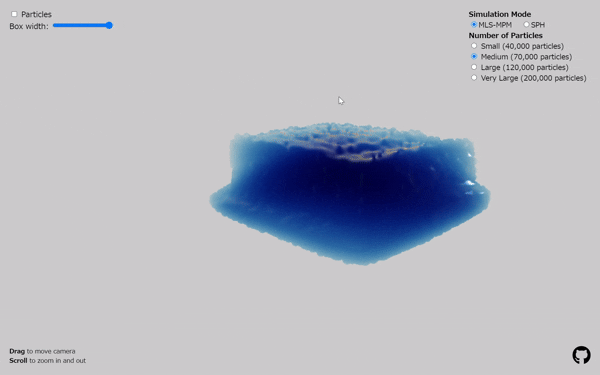
The second one is WaterBall (GitHub Repository):

After reading this article, I think you will have a better understanding of what is happening inside these simulations.
Fluid Simulation: Physics
There are many different methods for simulating fluids, generally categorized into three approaches.
Particle-based approaches
- Fluids are represented as a collection of particles, with their physical behavior simulated through the movement of these particles.
- Examples: SPH (Smoothed Particle Hydrodynamics), MPS (Moving Particle Semi-Implicit) method
Grid-based approaches
- Fluids are simulated using a grid that divides space into small, uniform cells. These approaches are particularly effective for simulating gases.
- Examples: VOF (Volume of Fluid) method
Hybrid approaches combining particle-based and grid-based methods
- Some methods integrate both particles and grids to leverage the advantages of each.
- Examples: FLIP (Fluid Implicit Particle), MPM (Material Point Method)

Here, I will explain SPH, a classical particle-based approach, and MLS-MPM, a relatively new hybrid approach. Please note that this will be a very brief explanation for simplicity. If you want to learn more, I recommend watching Coding Adventure: Simulating Fluids for SPH and reading the MPM Guide for (MLS-)MPM.
SPH (Smoothed Particle Hydrodynamics)
SPH (Smoothed Particle Hydrodynamics) is a well-known particle-based method for fluid simulation, where the fluid is represented as a collection of particles. Since its introduction to interactive fluid simulation in the seminal paper Particle-Based Fluid Simulation for Interactive Applications by Müller et al. in 2003, SPH has been widely used in many applications.
A single simulation step of SPH proceeds as follows:
- Calculate the density and pressure of each particle by summing the densities of nearby particles within a fixed distance.
- Compute forces such as pressure force and viscosity force by summing the contributions of nearby particles. External forces are also included in this step.
- Perform integration using the forces calculated in the previous step and update the particle positions accordingly.
The most computationally expensive procedure in the SPH method is the neighborhood search, which identifies nearby particles. A brute-force search requires quadratic time relative to the number of particles, making it very slow. To accelerate this process, various optimization techniques have been proposed. Using WebGPU, I implemented a fast neighborhood search algorithm on the GPU based on the approach presented in the slide FAST FIXED-RADIUS NEAREST NEIGHBORS: INTERACTIVE MILLION-PARTICLE FLUIDS. This significantly improved the nearest neighbor search performance compared to brute-force methods, enabling real-time simulation of approximately 30,000 particles on an iGPU.
However, I was not satisfied with this performance since my goal was to match the efficiency of Fluid Particles by David Li, which achieves real-time simulation of around 100,000 particles on an iGPU on my laptop. After further investigation, I discovered that this implementation uses the PIC/FLIP method, a hybrid approach combining particle-based and grid-based techniques. I also realized that the primary reason for its superior performance (as well as other hybrid approaches) is that it is completely free from neighborhood searches, which was a major bottleneck in my SPH implementation.
Therefore, I decided to shift toward implementing a hybrid approach to achieve higher simulation performance. Since implementing PIC/FLIP correctly seemed quite challenging, I opted for a different hybrid method, which I will explain below: MLS-MPM (Moving Least Squares Material Point Method).
MLS-MPM (Moving Least Squares Material Point Method)
MPM (Material Point Method) is an algorithm for simulating various materials that can be modeled using a constitutive equation. In MPM, a material is represented as a collection of small particles called “material points.” These material points do not interact directly but instead influence each other indirectly through a background grid.
It is well known that Disney used this algorithm for snow simulation in the movie Frozen, showcasing how effectively MPM can handle a wide range of materials.
MLS-MPM (Moving Least Squares Material Point Method), proposed by Hu et al. in 2018, is a faster version of MPM (paper here). This is the simulation algorithm I implemented for WebGPU-Ocean and WaterBall to achieve high-performance simulation.
Like other hybrid approaches, MPM and MLS-MPM simulate the movement of particles by repeatedly transferring physical quantities between particles and the grid—first by scattering data from particles to the grid (P2G stage), then gathering it back from the grid to the particles (G2P stage). Crucially, these algorithms do not require neighborhood searches, since information is exchanged via the grid rather than through direct particle-to-particle interactions, as seen in particle-based methods. As a result, these algorithms are expected to be significantly faster than SPH, where neighborhood search was a major bottleneck in my implementation.
Now, let’s examine how MLS-MPM, implemented in WebGPU, improves simulation performance compared to SPH. The simulation runs on an iGPU on an AMD Ryzen 7 5825U.


The difference is obvious! At last, we’ve achieved real-time simulation of 100,000 particles on an iGPU, thanks to MLS-MPM.
Fluid Simulation: Rendering
Now that we have a fast fluid simulation, let’s move on to rendering the fluid. Broadly, there are two main methods for real-time fluid rendering based on particle data: Marching Cubes and Screen-Space Fluid Rendering. Below is a brief overview of these methods.
Marching Cubes
- A mesh representing the fluid surface is generated from the particle data and used for rendering.
- Pros: The computational cost remains relatively unaffected by increases in rendering resolution.
- Cons: Low mesh resolution can result in visual artifacts.
Screen-Space Fluid Rendering
- Unlike Marching Cubes, this method performs the entire rendering process in screen space without constructing a mesh.
- Pros: Enables the real-time generation of a smooth and clean fluid surface.
- Cons: Computational cost increases rapidly with higher rendering resolution.

For WebGPU-Ocean and WaterBall, I chose Screen-Space Fluid Rendering as the rendering method. This decision was primarily influenced by the stunning rendering results showcased in the GDC 2010 slide. Below, I provide a more detailed explanation of this method.
Screen-Space Fluid Rendering
Screen-Space Fluid Rendering (abbreviated as SSFR below) is a relatively new technique for rendering fluids in real-time. In SSFR, the entire rendering process is performed in screen space without generating a mesh. Each step of SSFR is described below. (Note: For a more in-depth understanding of this method, I recommend reading the GDC 2010 slide and the Technical Implementation Details in the Babylon.js documentation.)
Step 1: Render the depth of each particle
Step 2: Render the thickness of each particle using additive blending

Step 3: Apply smoothing to the depth map and thickness map using filters such as the Bilateral Filter and Gaussian Filter.
Step 4: Compute surface normals from the smoothed depth map.

Step 5: Using surface normals, compute reflection, refraction, and specular lighting to render the final result.
The thickness calculated in the previous step can be used for refraction calculations.

In my opinion, fluid rendered using SSFR appears very clean and visually appealing! If high-resolution output is not a requirement, this method is an excellent choice for fluid rendering.
A drawback of SSFR is that its computational cost increases rapidly with higher rendering resolutions. To mitigate this, I limit the rendering resolution to half the window size in WaterBall. Additionally, saharan (@shr_id) mentioned in their article (Japanese) that they use Marching Cubes instead of SSFR for fluid rendering in the VRChat World due to the need for high-resolution rendering.
First Project: WebGPU-Ocean
Now, let’s return to my first fluid simulation implemented in WebGPU: WebGPU-Ocean.

By now, you can probably guess how this is implemented—yes, the simulation algorithm is MLS-MPM, and the fluid is rendered using Screen-Space Fluid Rendering. You can toggle between simulation algorithms to compare the performance differences between MLS-MPM and SPH. (Note: On Mac, switching to SPH appears to freeze the simulation.)
Second Project: WaterBall
As a follow-up project to WebGPU-Ocean, I implemented the next fluid simulation: WaterBall.

The inspiration for this project came from the video below. It made me really want to create something similar that allows real-time interaction!
The good news is that this simulation runs smoothly even on my six-year-old iPad Air 3!
The simulation algorithm and rendering method are essentially the same as in WebGPU-Ocean, but I implemented some rendering enhancements to make it look more visually striking.
Adjust particle size based on density
- In the standard implementation of SSFR, all particles are rendered at the same size. However, this appears somewhat unnatural, as real water droplets vary in size.
- To improve realism, I modify each particle’s size based on its density obtained from the simulation, making isolated particles appear smaller.

Stretch particles in the direction of their velocity
- Rendering all particles as circles works well, but stretching them based on their velocity adds a sense of motion and makes the simulation appear more dynamic.

Edging
- When rendering the fluid, edges are highlighted at depth discontinuities. This enhances the visibility of fluid flow and droplets, making the motion more distinct.

I learned these techniques by closely observing simulations and studying the source code by saharan (@shr_id), a master of real-time physics simulation in browsers. A huge thanks to them!
Conclusion
By combining MLS-MPM for simulation and Screen-Space Fluid Rendering for visualization, it’s possible to achieve real-time, high-quality fluid simulations in the browser. WebGPU makes this approach both efficient and practical, allowing complex physics to run smoothly even on integrated graphics.
- MLS-MPM (Moving Least Squares Material Point Method), a hybrid approach combining particle-based and grid-based methods, was used for high-performance fluid simulation. By implementing this method with WebGPU, real-time simulation of ~100,000 particles on an iGPU is now possible.
- Screen-Space Fluid Rendering was employed to render a clean and dynamic fluid surface.
- Using MLS-MPM and Screen-Space Fluid Rendering, I developed the following fluid simulations:
In my opinion, thanks to compute shader support in WebGPU, implementing physics simulations for browsers using GPGPU has become more accessible than ever. For instance, implementing the P2G stage in MPM using WebGL can be challenging, as it requires additive blending to scatter particle data to the grid. In contrast, WebGPU makes this process more intuitive by providing atomicAdd
in the compute shader. Similarly, I believe many other simulation algorithms have become easier to implement thanks to WebGPU.
Currently, WebGPU support is still limited in some browsers. However, as adoption grows, we can expect to see even more exciting physics simulations running in the browser. Hopefully, one day, browser-based physics simulations will rival those created with powerful engines like Unreal Engine!